Split component
The main purpose of the Split component is to split single messages into multiple messages based on a configured expression. The splitted messages are sent out of the bottom output of the component.
The Aggregation configuration defines if the input message or an aggregated message is sent out of the right output of the Split component.
Configuration
The Split component has the following configuration options:
Expression
The expression that defines how to split the incoming message.
Type
The type of expression you are defining. See Using Split - Expressions on this page for examples.
Options
Click on the expression types above to navigate to the respective reference page with examples.
Use namespace
Enable this option to define a namespace if it is used in an XML.
Namespace prefix
The prefix of the namespace that is used in the XML.
Only available when Use namespace is enabled.
Namespace
The namespace that is used in the XML.
Only available when Use namespace is enabled.
Aggregation
Defines if you want to aggregate the splitted messages, and if so, the data format of message you want to aggregate. See Using Split - Aggregation on this page for examples.
Options
None
(default)XML
JSON
Select None
if you don't want to aggregate the splitted messages. The input message is sent out of the right output of the Split component.
Select XML
or JSON
to aggregate the splitted messages into one message after following the flow on the bottom output. The aggregated message is sent out of the right output of the Split component.
Use streaming
Enable this option to split the input message into chunks to reduce memory overhead when dealing with large files.
Because the splitting is done in chunks the total amount of splitted messages is only known when the last message is being splitted. Read more about Headers on splitted messages.
Split parallel
Enable this option to split each message concurrently. Note that the component will still wait until all messages have been fully processed before it continues. It's only the processing of the splitted messages that happens concurrently.
Parallel splitting may cause a severe increase of system usage, such as CPU, as everything happens concurrently. We do not recommend to enable this option when splitting large files.
Exchange pattern
This option determines how the messages are sent to the bottom and right output.
Options
One way
Request reply
(default)
One way
One way
means that the Split component will do the splitting in a asynchronous way so order is not guaranteed. You want to use this option when:
- The order of the splitted messages doesn't matter.
- You don't want to wait for the splitting process to finish before the input message is sent out of its right output.
- Each splitted message goes through a lot of logic. For example: an outbound flow link that connects to a big flow which in turn connects to another big flow.
Regardless wether the Exchange pattern is set to One way
, when Aggregation is set to XML
or JSON
, the Split component has to wait for the splitting process to finish before the aggregated message is sent out of its right output.
Request reply
Request reply
is the opposite of the One way
option. This sequential way of splitting will guarantee the order of the splitted messages.
When Aggregation is set to None
the input message is sent out of the right output of the Split component after the splitting process is finished.
When using Request reply
you might need to increase the Components timeout option of a flow to accomodate for the time it takes for the splitting process to finish.
Headers on splitted messages
The Split component adds a couple of headers to splitted messages. They are available on each splitted message that's sent out of the bottom output and can be used during the splitting process, for example to monitor the process.
Header | Type | Description |
---|---|---|
CamelSplitIndex | int | A counter that increases for each message being split. It starts from 0 . |
CamelSplitSize | int | The total number of messages that are splitted. |
CamelSplitComplete | boolean | Whether or not the splitted message is the last. |
When Use Streaming is enabled the CamelSplitSize
header only has a value on the last splitted message.
Using Split - Expression types
Simple
Input message: collection of Java objects
Expression: ${bodyAs(String)}
Bottom output messages: one message for each Java Object
Splitting messages using the given Simple expression can be useful if you've constructed a message containing a collection of Java objects using the Script component.
XPath
Input message:
<bookstore>
<book title="one"/>
<book title="two"/>
</bookstore>
Expression: //book
Bottom output messages: <book title="one"/>
and <book title="two"/>
XPath (dynamic)
By using a header within an XPath expression you can split an XML based on the value of an element's attribute.
Input message:
<?xml version="1.0" encoding="utf-8"?>
<orders>
<order attribute="1">
<data>1</data>
<data>2</data>
<data>3</data>
</order>
<order attribute="2">
<data>a</data>
<data>b</data>
<data>c</data>
</order>
</orders>
Expression: //order[@attribute=$in:split_header]
The headername used to split the XML dynamically is split_header
.
Bottom output messages:
<order attribute="1">
<data>1</data>
<data>2</data>
<data>3</data>
</order>
<order attribute="2">
<data>a</data>
<data>b</data>
<data>c</data>
</order>
Not setting a split_header
header at all or setting it to anything but 1
or 2
will not split the XML.
No message will come out under the split and the XML won't be 'encapsulated' within the <Aggregated>
element when aggregation is set.
Tokenizer
Input message: one@two@three
Expression: @
Bottom output messages: one
, two
and three
Using Split - Aggregation
To show how Aggregation works see the example below. We use XPath to split an XML and use the splitted messages as input for an HTTP request.
The two sections below explain what happens at the right output of the Split component after the splitted messages are sent out of the bottom output without Aggregation and with Aggregation.
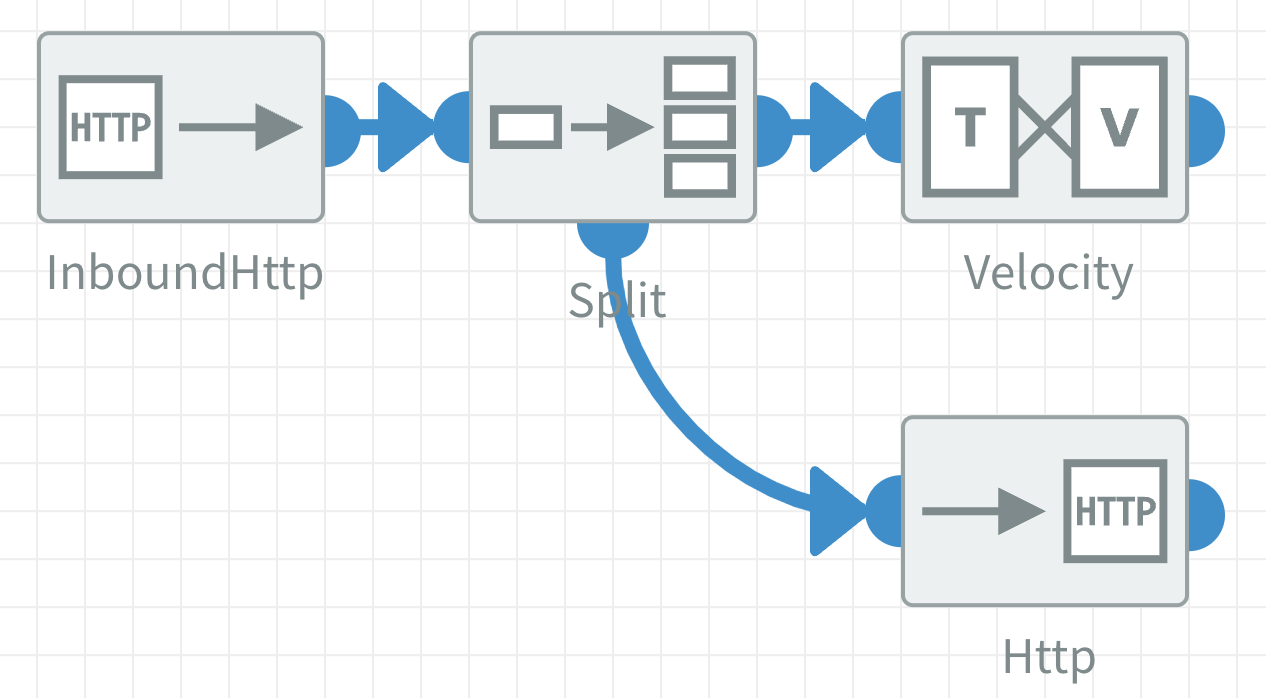
Input message:
<bookstore>
<book title="one"/>
<book title="two"/>
</bookstore>
Expression: //book
Bottom output messages: <book title="one"/>
and <book title="two"/>
Without Aggregation
When Aggregation is set to None
each splitted message is sent out of the bottom output to the HTTP component. The response of the HTTP request is not 'returned' to the Split component. This makes it ideal for POST requests to an HTTP endpoint.
The message that's sent to the right output of the Split component is the same as the input message.
Right output message:
<bookstore>
<book title="one"/>
<book title="two"/>
</bookstore>
The Exchange pattern configuration defines if the message is sent to the right output directly after it has been received (One way
) or only after the Split component finishes the splitting process (Request reply
).
With Aggregation
When Aggregation is set to XML
or JSON
each splitted message is sent out of the bottom output to the HTTP component. The response of the HTTP request is 'returned' to the Split component. This makes it ideal for GET requests to an HTTP endpoint to retrieve additional data.
Responses HTTP request:
<book_data>
<title>one</title>
<author>john</author>
<price>10.50</price>
</book_data>
<book_data>
<title>two</title>
<author>doe</author>
<price>7.50</price>
</book_data>
The Split component will aggregate the splitted messages, in this case the response of the HTTP request, into one message that is sent out of its right output to the Velocity component.
Right output message:
<?xml version="1.0" encoding="UTF-8"?>
<Aggregated>
<book_data>
<title>one</title>
<author>john</author>
<price>10.50</price>
</book_data>
<book_data>
<title>two</title>
<author>doe</author>
<price>7.50</price>
</book_data>
</Aggregated>
The Split component can only aggregate messages with the data format that is set in the Aggregation configuration. For instance, when XML
is configured the last component in the bottom route needs to produce a valid XML to be able to aggregate it. Invalid messages are ignored and not aggregated.
With Aggregation and Filter component
Using a Filter component in the bottom route of the Split component with Aggregation set to XML
or JSON
can give unexpected results. See the example below:
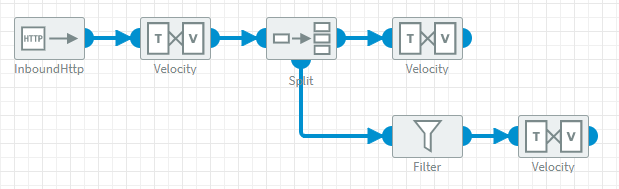
Input message:
<?xml version="1.0" encoding="utf-8"?>
<orders>
<order>
<ordernr>1</ordernr>
</order>
<order>
<ordernr>2</ordernr>
</order>
</orders>
Expression: //order
Expression Filter component: /order/ordernr/text() != '1'
The Velocity component after the Split component contains this message body:
<orderNew>
<ordernr>New</ordernr>
</orderNew>
Right output message:
<Aggregated>
<order>
<ordernr>1</ordernr>
</order>
<orderNew>
<ordernr>New</ordernr>
</orderNew>
</Aggregated>
When the expression in the Filter component is not met, the message entering it is 'returned' for aggregation.
There is an alternative solution if this is not the behaviour you are looking for. Instead of the Filter component use a Content Router component with the same XPath expression and the same Velocity component as before. Then add a Velocity component to the otherwise
route with this message body:
<orderNew/>
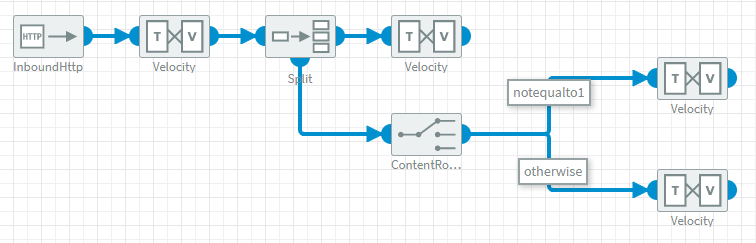
Right output message:
<Aggregated>
<orderNew/>
<orderNew>
<ordernr>New</ordernr>
</orderNew>
</Aggregated>
Now when the expression defined in the Content Router component is not met, the newly formatted message is 'returned' for aggregation.
Nested Splits with Aggregation
In the example below there are two nested Split components.
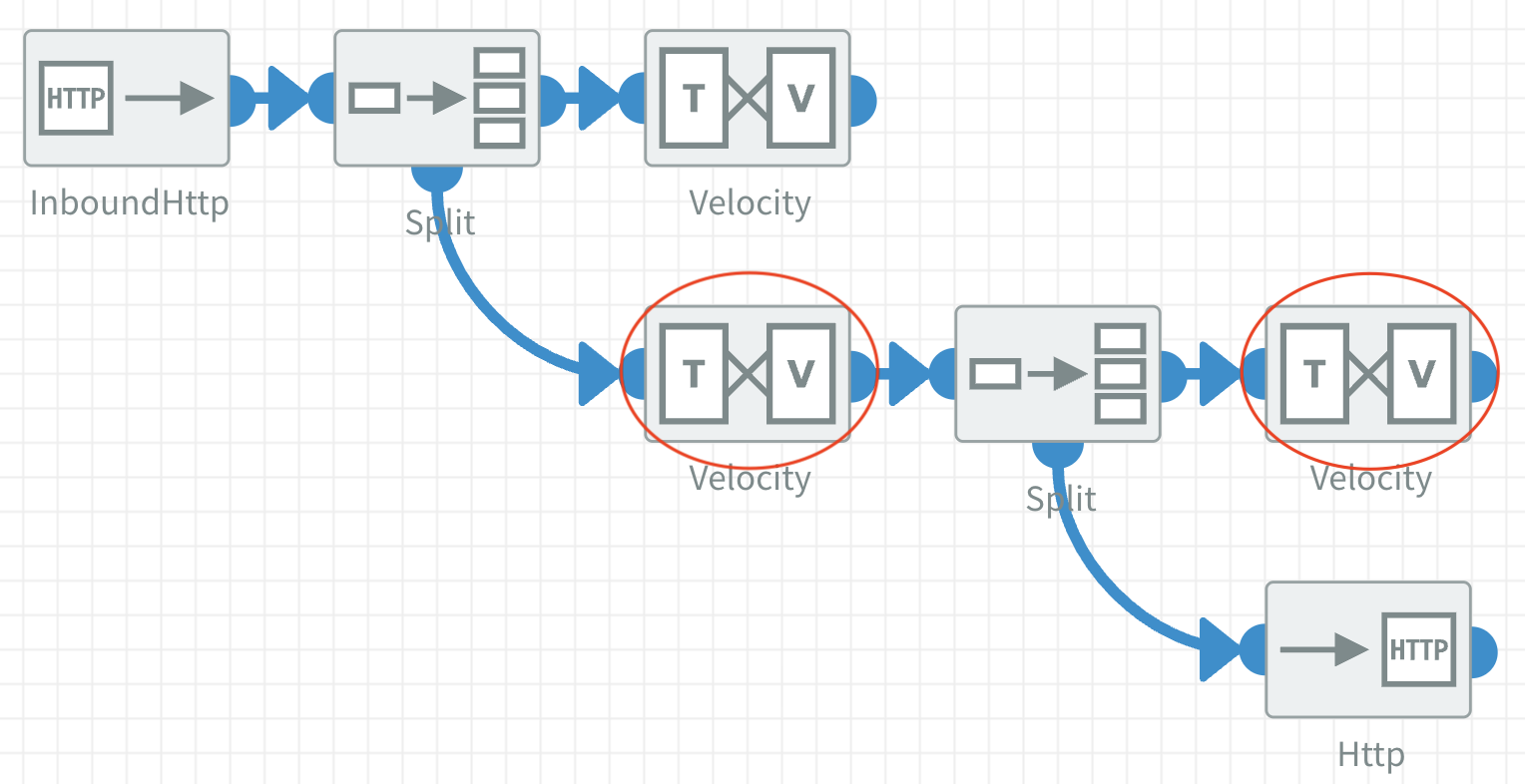
For aggregation to work in this example make sure to add Velocity components in the cirled locations that contain this message body:
${bodyAs(String)}